Infinite scroll is everywhere you look. Twitter, Facebook and Pinterest are probably the most famous examples. It became popular with the introduction of real-time streams, as you come across on social networks. But now a days you see it everywhere, from e-commerce websites to Single Page Applications.
In this article we will explore some common pitfalls with infinite scroll implementations and provide answers on how you can avoid them using Infinite Ajax Scroll.
TL;DR: Infinite Ajax Scroll can help us with (1) keeping our footer reachable, (2) not breaking the back button and (3) optimizing for SEO.
1. Unreachable footer
A common problem with infinite scrolling pages is when a footer is unreachable. A footer often contains important information visitors are interested in, like contact information. Visitors will become frustrated when they can't reach it due to a faulty infinite scroll implementation.
To solve this issue a number of solutions come to mind.
Solution A: Sticky footer
Okay, this feels a bit like cheating, but nonetheless it's very effective. In stead of having the important links in a footer at the bottom of the page, we can place them in a sidebar. A great example of this is Twitter. Their "footer" links are in a fixed sidebar. So no matter how far a user scrolls, the links are always available.
Instead of putting the footer links in a sidebar, we could also make the footer itself sticky.
Solution B: Add a "Load more" button after 1 or more pages
This is probably the most common solution. For the first one or two pages we enable infinite scroll, after that we show a "Load More" button. This solution is simple and effective.
To build our solution we can take advantage of Infinite Ajax Scroll's page
event.
import InfiniteAjaxScroll from '@webcreate/infinite-ajax-scroll';
window.ias = new InfiniteAjaxScroll('.container', {
// configuration
});
ias.on('page', (e) => {
// from page 2 onwards we stop automatic loading of the next page
if (e.pageIndex >= 2) {
this.disableLoadOnScroll();
// @todo show load more button and restore loadOnScroll (not yet available during beta)
}
});
Solution C: Measure scroll speed and adjust accordingly
This is a more advanced solution and builds on this hypothesis: When a user scrolls fast to the bottom of the page, (s)he probably wants to reach the footer.
So if we determine, based on the scroll speed, that the user wants to reach the footer, we disable the automatic loading of the next page and show a "Load More" button.
Slow scrolling = automatic loading of next page
Fast scrolling = "Load More" button
The complex part here is the scroll tracking. Luckily Infinite Ajax Scroll provides this information with the scrolled
event. Let's see how we can utilize this:
import InfiniteAjaxScroll from '@webcreate/infinite-ajax-scroll';
window.ias = new InfiniteAjaxScroll('.container', {
// configuration
});
ias.on('scrolled', (e) => {
// high delta means high scroll velocity
// @todo 300 is arbitrary and needs some experimentation
if (e.scroll.deltaY > 300) {
// disable automatic loading of next page
this.disableLoadOnScroll();
}
// @todo show load more button and restore loadOnScroll (not yet available during beta)
});
2. Breaking the back button
Another problem with infinite scroll can arise when the history isn't updated when the user scrolls through the pages. Say a user scrolled to page three and clicks an article link. After reading the article the user wants to go back to the list to read another article. Without history support the user would return to page one. Of course most users would now leave.
Not only does this break the back button, but it also prevents users from sharing the url to the current page. Normally a user can copy the url from the browser and bookmark it, or share it with others. Without history support this is not an option, as the url always stays the same, no matter to which page the user has scrolled.
Infinite Ajax Scroll solves this issue. It constantly keeps track of the current page and emits a page
event on change. As a developer we can hook into this event and update the url and browser title:
import InfiniteAjaxScroll from '@webcreate/infinite-ajax-scroll';
window.ias = new InfiniteAjaxScroll('.container', {
// configuration
});
// update title and url then scrolling through pages
ias.on('page', (e) => {
document.title = e.title;
let state = history.state;
history.replaceState(state, e.title, e.url);
});
You can see this behaviour in our blog example. As we scroll between pages the browser url is automatically updated to match the current page.
3. SEO unfriendly
An import factor to keep in mind while implementing infinite scroll is its impact on SEO. If we build an infinite scroll without a fallback to a non-javascript pagination, we could negatively affect our SEO ranking.
Google has a great article about this. Some of its recommendations include:
- Make sure your pages can be accessed when JavaScript is disabled
- Keep reasonable content length and page load times per page
- Chunk your pages without overlap
- Structure urls correctly for search engine processing
- Use the browsers History API when scrolling through pages
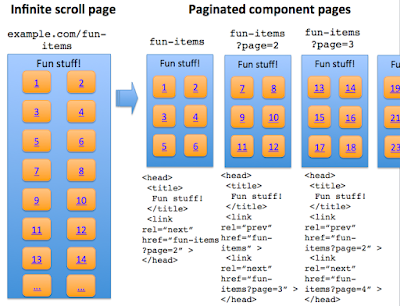
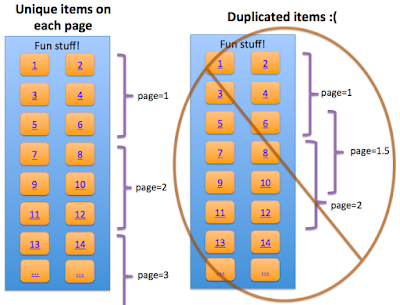
Point 5 can easily be solved by Infinite Ajax Scroll (see previous pitfall), points 1-4 we have to keep in mind when building our (server-side) pagination.